Thought I'd start a new thread on TA (Technical Analysis) functions written in "C" that can be used by other 32 bit software. The 32 bit software I hopefully demonstrate in later posts are 32 bit Perl, Excel 2003 and Metatrader 4, but probably could be extended to say 32 bit versions of Python, Ruby, Maple, Mathematica, R, Metastock, Amibroker, MathLab, WealthLab etc. Creating a library is useful because you should expect the same output results given the same input data for say a moving average no matter what software calls your functions.
What I've done is written some basic moving average "C" code (SMA,EMA,SMMA,LWMA) using the free codeblocks IDE (Integrated Development Environment) that comes with the free C/C++ compiler and used this for my library. In later posts, I explain in detail, the steps involved in creating libraries for use with other software. These steps are:
1) Install codeblocks IDE with free C/C++ compiler
2) Start a new project and compile "C" files written by me to create a 32 bit "C" static link library called "libtal.a"
3) Create a "C++" test project (console application) with "main.cpp" that links to the library functions created in "libtal.a"
4) Create a 32 bit Windows DLL (dynamic link library) that links to the library functions created in "libtal.a"
5) Test 32 bit Windows DLL with 32 bit software packages Perl and Excel 2003.
6) Test 32 bit Windows DLL with MT4 (Metatrader 4).
7) Creating a 32 bit Amibroker DLL that links to my library functions created in libtal.a
Special Note: All work done in the 6 steps described above was carried out on a 32 bit Windows XP platform.
1) Installing codeblocks IDE with free C/C++ compiler
To start off with, you will need to go to the following website
http://www.codeblocks.org/
Navigate to downloads/binaries and download the latest codeblocks with mingw. As of 23/Feb/2015, the latest is 13.12 and this is the one I'll be using for this thread. So now we download the file "codeblocks-13.12mingw-setup.exe" and install codeblocks. This installation contains both the IDE and the free 32 bit C/C++ compiler. The 32 bit C/C++ compiler is what is needed to compile my project code.
When you have successfully installed codeblocks, it should look something like this when you open it up:
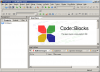
What I've done is written some basic moving average "C" code (SMA,EMA,SMMA,LWMA) using the free codeblocks IDE (Integrated Development Environment) that comes with the free C/C++ compiler and used this for my library. In later posts, I explain in detail, the steps involved in creating libraries for use with other software. These steps are:
1) Install codeblocks IDE with free C/C++ compiler
2) Start a new project and compile "C" files written by me to create a 32 bit "C" static link library called "libtal.a"
3) Create a "C++" test project (console application) with "main.cpp" that links to the library functions created in "libtal.a"
4) Create a 32 bit Windows DLL (dynamic link library) that links to the library functions created in "libtal.a"
5) Test 32 bit Windows DLL with 32 bit software packages Perl and Excel 2003.
6) Test 32 bit Windows DLL with MT4 (Metatrader 4).
7) Creating a 32 bit Amibroker DLL that links to my library functions created in libtal.a
Special Note: All work done in the 6 steps described above was carried out on a 32 bit Windows XP platform.
1) Installing codeblocks IDE with free C/C++ compiler
To start off with, you will need to go to the following website
http://www.codeblocks.org/
Navigate to downloads/binaries and download the latest codeblocks with mingw. As of 23/Feb/2015, the latest is 13.12 and this is the one I'll be using for this thread. So now we download the file "codeblocks-13.12mingw-setup.exe" and install codeblocks. This installation contains both the IDE and the free 32 bit C/C++ compiler. The 32 bit C/C++ compiler is what is needed to compile my project code.
When you have successfully installed codeblocks, it should look something like this when you open it up:
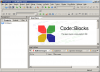